The estimated reading time 5 minutes
I frequently receive requests from customers who are dealing with the default sharing settings within the Exchange organization and don’t have a reasonable solution for it. Therefore, I sat down and wrote a small PowerShell script that can initially read the default permissions of all user mailboxes.
In this case, default permissions refer to the permissions that everyone initially has; the default is ‘Availability Only’. So, by default, you can only see Free/Busy.
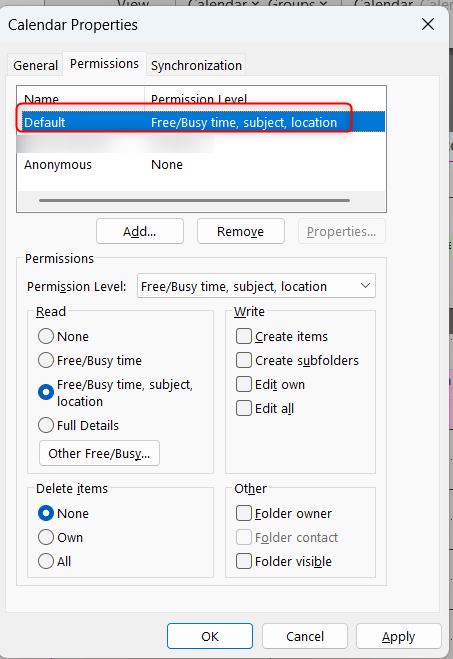
Currently, this cannot be centrally controlled when creating accounts (unless you create the mailboxes directly via PowerShell, see a post on this).
With the following script, you can read the mentioned default permissions for all active mailboxes (excluding shared mailboxes or resource mailboxes) and export them to a CSV file.
Such a CSV file should be created. To make the assignment easier, the display name and email address are also output here:
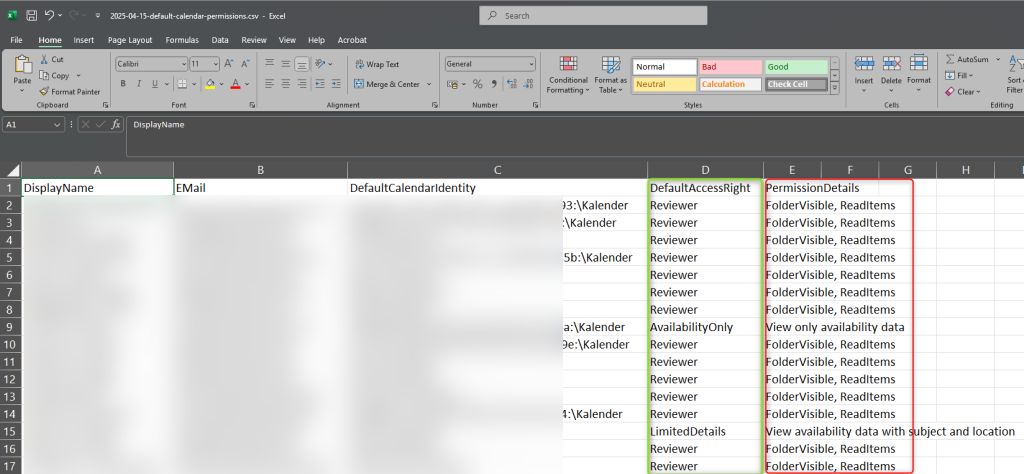
The default permission on the mailbox calendar is outlined in green, while a detailed list of the permission roles is outlined in red.
Here is a small tutorial on Exchange Online PowerShell.
In the script, the path ($outputpath) for the CSV output must be adjusted, and a connection to Exchange Online PowerShell must already be established.
NOTE: A language query has been built in, as the calendar folders are called ‘Kalender’ in German and ‘Calendar’ in English. Other languages are not considered here but can be added to the switch case function at any time (line 24).
### connect to Exchange Online!!! #Connect-ExchangeOnline #set path for output $outputpath = "C:\temp\2025-04-15-default-calendar-permissions.csv" ##### begin of the script $alias = (get-mailbox -RecipientTypeDetails UserMailbox -ResultSize unlimited).alias | Sort-Object #defining csv format "DisplayName;EMail;DefaultCalendarIdentity;DefaultAccessRight;PermissionDetails" | Out-File -FilePath "$outputpath" -Encoding utf8 -Force #looping through mailboxes foreach($user in $alias){ #getting more user properties $userprops = get-user $user $displayname = ($userprops).DisplayName $email = ($userprops).WindowsEmailAddress #show status in powershell output Write-Host "Checking $displayname,$email" -ForegroundColor Yellow #check language $language = (Get-MailboxRegionalConfiguration $user).language.name switch($language){ de-DE {$ident = "$user" + ":\Kalender"} en-US {$ident = "$user" + ":\Calendar"} Default {$ident = "$user" + ":\Calendar"} } #getting permissions $calendardefaultperms = Get-MailboxFolderPermission -Identity "$ident" | Where-Object{$_.user -match "Default"}| Select-Object Identity,Foldername,User,AccessRights $calendarident = ($calendardefaultperms).Identity $calendarperms = $calendardefaultperms.AccessRights #list detailed permission for roles switch($calendarperms){ Author {{$permsdetails ="CreateItems, DeleteOwnedItems, EditOwnedItems, FolderVisible, ReadItems"}} Contributor {$permsdetails ="CreateItems, FolderVisible"} Editor {$permsdetails ="CreateItems, DeleteAllItems, DeleteOwnedItems, EditAllItems, EditOwnedItems, FolderVisible, ReadItems"} NonEditingAuthor {$permsdetails ="CreateItems, DeleteOwnedItems, FolderVisible, ReadItems"} Owner {$permsdetails ="CreateItems, CreateSubfolders, DeleteAllItems, DeleteOwnedItems, EditAllItems, EditOwnedItems, FolderContact, FolderOwner, FolderVisible, ReadItems"} PublishingAuthor {$permsdetails ="CreateItems, CreateSubfolders, DeleteOwnedItems, EditOwnedItems, FolderVisible, ReadItems"} PublishingEditor {$permsdetails ="CreateItems, CreateSubfolders, DeleteAllItems, DeleteOwnedItems, EditAllItems, EditOwnedItems, FolderVisible, ReadItems"} Reviewer {$permsdetails ="FolderVisible, ReadItems"} AvailabilityOnly {$permsdetails ="View only availability data"} LimitedDetails {$permsdetails ="View availability data with subject and location"} Default {$permsdetails ="individual permissions no role set"} } #summarize information for csv export "$displayname;$email;$calendarident;$calendarperms;$permsdetails" | Out-File -FilePath "$outputpath" -Encoding utf8 -Append ###change default permissions for ALL mailboxes, be careful! #set-MailboxFolderPermission -Identity "$iden" -User Default -AccessRights Reviewer }
With this script, it is also possible to adjust all mailboxes to a ‘standard’ (of course, only existing ones; future ones would also need to be adjusted with this script).
To adjust the default permission, the comment in line 50 must be removed, so instead of ‘#set-MailboxFolderPermission -Identity “$ident” -User Default -AccessRights Reviewer‘, it should be ‘set-MailboxFolderPermission -Identity “$ident” -User Default -AccessRights Reviewer’.

The complete script looks as follows.
NOTE: The script changes the permission on all mailboxes to the permission defined in the script, see also permission roles
### connect to Exchange Online!!! #Connect-ExchangeOnline #set path for output $outputpath = "C:\temp\2025-04-15-default-calendar-permissions.csv" ##### begin of the script $alias = (get-mailbox -RecipientTypeDetails UserMailbox -ResultSize unlimited).alias | Sort-Object #defining csv format "DisplayName;EMail;DefaultCalendarIdentity;DefaultAccessRight;PermissionDetails" | Out-File -FilePath "$outputpath" -Encoding utf8 -Force #looping through mailboxes foreach($user in $alias){ #getting more user properties $userprops = get-user $user $displayname = ($userprops).DisplayName $email = ($userprops).WindowsEmailAddress #show status in powershell output Write-Host "Checking $displayname,$email" -ForegroundColor Yellow #check language $language = (Get-MailboxRegionalConfiguration $user).language.name switch($language){ de-DE {$ident = "$user" + ":\Kalender"} en-US {$ident = "$user" + ":\Calendar"} Default {$ident = "$user" + ":\Calendar"} } #getting permissions $calendardefaultperms = Get-MailboxFolderPermission -Identity "$ident" | Where-Object{$_.user -match "Default"}| Select-Object Identity,Foldername,User,AccessRights $calendarident = ($calendardefaultperms).Identity $calendarperms = $calendardefaultperms.AccessRights #list detailed permission for roles switch($calendarperms){ Author {{$permsdetails ="CreateItems, DeleteOwnedItems, EditOwnedItems, FolderVisible, ReadItems"}} Contributor {$permsdetails ="CreateItems, FolderVisible"} Editor {$permsdetails ="CreateItems, DeleteAllItems, DeleteOwnedItems, EditAllItems, EditOwnedItems, FolderVisible, ReadItems"} NonEditingAuthor {$permsdetails ="CreateItems, DeleteOwnedItems, FolderVisible, ReadItems"} Owner {$permsdetails ="CreateItems, CreateSubfolders, DeleteAllItems, DeleteOwnedItems, EditAllItems, EditOwnedItems, FolderContact, FolderOwner, FolderVisible, ReadItems"} PublishingAuthor {$permsdetails ="CreateItems, CreateSubfolders, DeleteOwnedItems, EditOwnedItems, FolderVisible, ReadItems"} PublishingEditor {$permsdetails ="CreateItems, CreateSubfolders, DeleteAllItems, DeleteOwnedItems, EditAllItems, EditOwnedItems, FolderVisible, ReadItems"} Reviewer {$permsdetails ="FolderVisible, ReadItems"} AvailabilityOnly {$permsdetails ="View only availability data"} LimitedDetails {$permsdetails ="View availability data with subject and location"} Default {$permsdetails ="individual permissions no role set"} } #summarize information for csv export "$displayname;$email;$calendarident;$calendarperms;$permsdetails" | Out-File -FilePath "$outputpath" -Encoding utf8 -Append ###change default permissions for ALL mailboxes, be careful! set-MailboxFolderPermission -Identity "$ident" -User Default -AccessRights LimitedDetails }
I hope I could shed some light on the default permissions within Exchange calendars. If you liked the article, feel free to press ‘Helpful’. For questions and suggestions, please use the comment function.